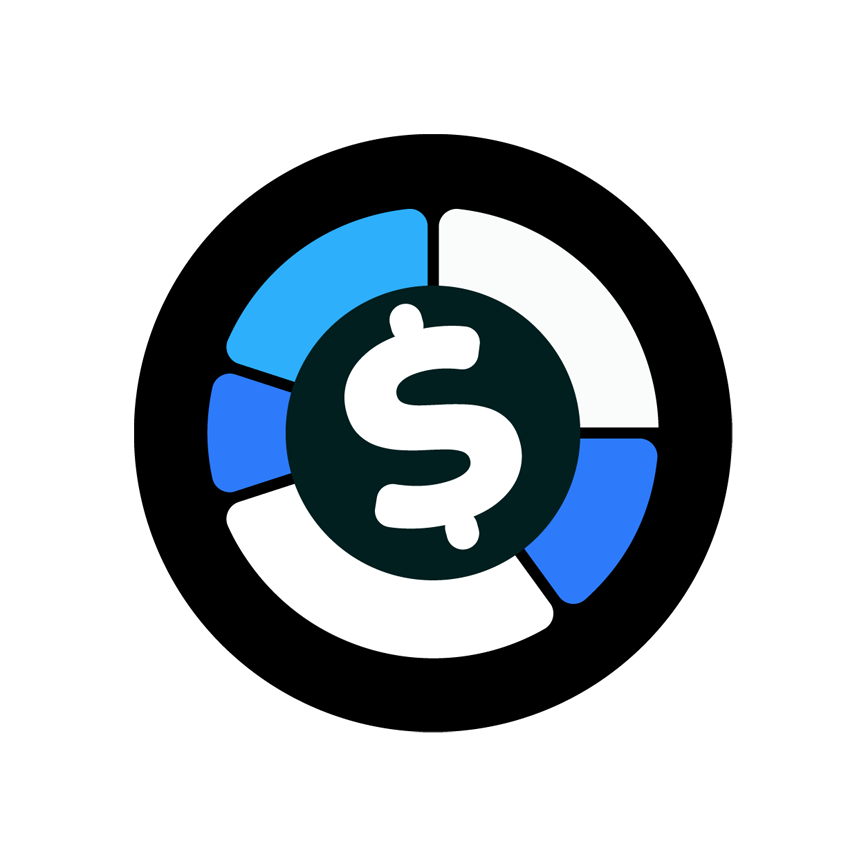
PPalytics
Project Overview
PPalytics is a powerful client-side web application that transforms PayPal transaction data into comprehensive business insights. Originally built as a personal tool, it evolved into a full-featured analytics platform that processes data entirely in the browser, ensuring complete privacy and security.
Key Features
12 Interactive Charts
Smart Processing
Tax Preparation
Core Capabilities
Pattern Detection
Customer Analytics
Financial Trends
Privacy Focus
Client-side Only
No Data Storage
Offline Support
Interactive Interface
Financial Analytics Dashboard
Comprehensive financial analysis with interactive visualizations:
Transaction Analysis
- Income vs expense tracking
- Transaction categorization
- Recurring payment detection
- Payment pattern analysis
Business Intelligence
- Customer retention metrics
- Growth trend analysis
- Seasonal patterns
- Revenue forecasting
Tax Preparation Tools
Financial Reports
- Quarterly breakdowns
- Annual summaries
- Category-based reporting
- Custom date ranges
Export Options
- Excel report generation
- PDF documentation
- Data visualization export
- Raw data access
System Architecture
Core Technologies
- Frontend: JavaScript, HTML5, CSS3
- Data Processing: CSV parsing and analysis
- Visualization: Chart.js
- Storage: Client-side only
Data Flow
- Input: PayPal CSV import
- Processing: Pattern detection algorithms
- Analysis: Transaction categorization
- Output: Interactive visualizations
Core Features
Smart Analytics
- Business detection algorithms
- Recurring payment identification
- Multi-currency support
- Customer retention tracking
Visualization Suite
- Income vs expense trends
- Customer growth patterns
- Seasonal analysis
- Transaction distributions
Tax Tools
- Quarterly breakdowns
- Category sorting
- Excel report generation
- Year-over-year analysis
Data Processing Pipeline
CSV Processing
- Automated format detection
- Data validation checks
- Currency normalization
- Transaction deduplication
Pattern Recognition
- Recurring payment detection
- Business name normalization
- Category classification
- Trend identification
Technical Implementation
Data Processing Pipeline
- CSV parsing and validation
- Transaction categorization algorithms
- Pattern recognition system
- Data normalization
Privacy & Security
- Client-side processing only
- No data storage or transmission
- Offline functionality
- Local file handling
Technical Implementation
Front-End Development
- Semantic HTML5 structure
- CSS3 with flexbox and grid
- JavaScript ES6+ features
- Responsive design patterns
Core Libraries
- Chart.js visualization suite
- HTML2PDF.js for reports
- XLSX.js CSV processing
- Custom data parsers
Technical Challenges
Performance Optimization
Processing large transaction datasets in browser
Solution:
- Implemented chunked processing
- Optimized data structures
- Added progressive loading
Data Standardization
Inconsistent PayPal transaction formats
Solution:
- Created format detection system
- Built data normalization pipeline
- Implemented error handling
Credits & Acknowledgements
This project was inspired by the needs of small business owners and freelancers who require better insights into their PayPal transaction data. Special thanks to the open-source community for the powerful libraries that made this project possible.
Built with Chart.js for visualizations, XLSX.js for CSV processing, and HTML2PDF.js for report generation. The project emphasizes privacy and security by processing all data client-side, ensuring user financial data never leaves their browser.